#!/usr/bin/env python
# coding: utf-8
#
Please cite us if you use the software
# # Chakraborty Dynamic Model
# ### Version 1.4
#
# ## Overview
#
# The new dynamic model is presented based on constant fuel utilization control (constant stoichiometry condition). The model solves the long-standing problem of mixing reversible and irreversible potentials (equilibrium and non-equilibrium states) in the Nernst voltage expression. Specifically, a Nernstian gain term is introduced for the constant fuel utilization condition, and it is shown that the Nernstian gain is an irreversibility in the computation of the output voltage of the fuel cell.
#
#
#
#
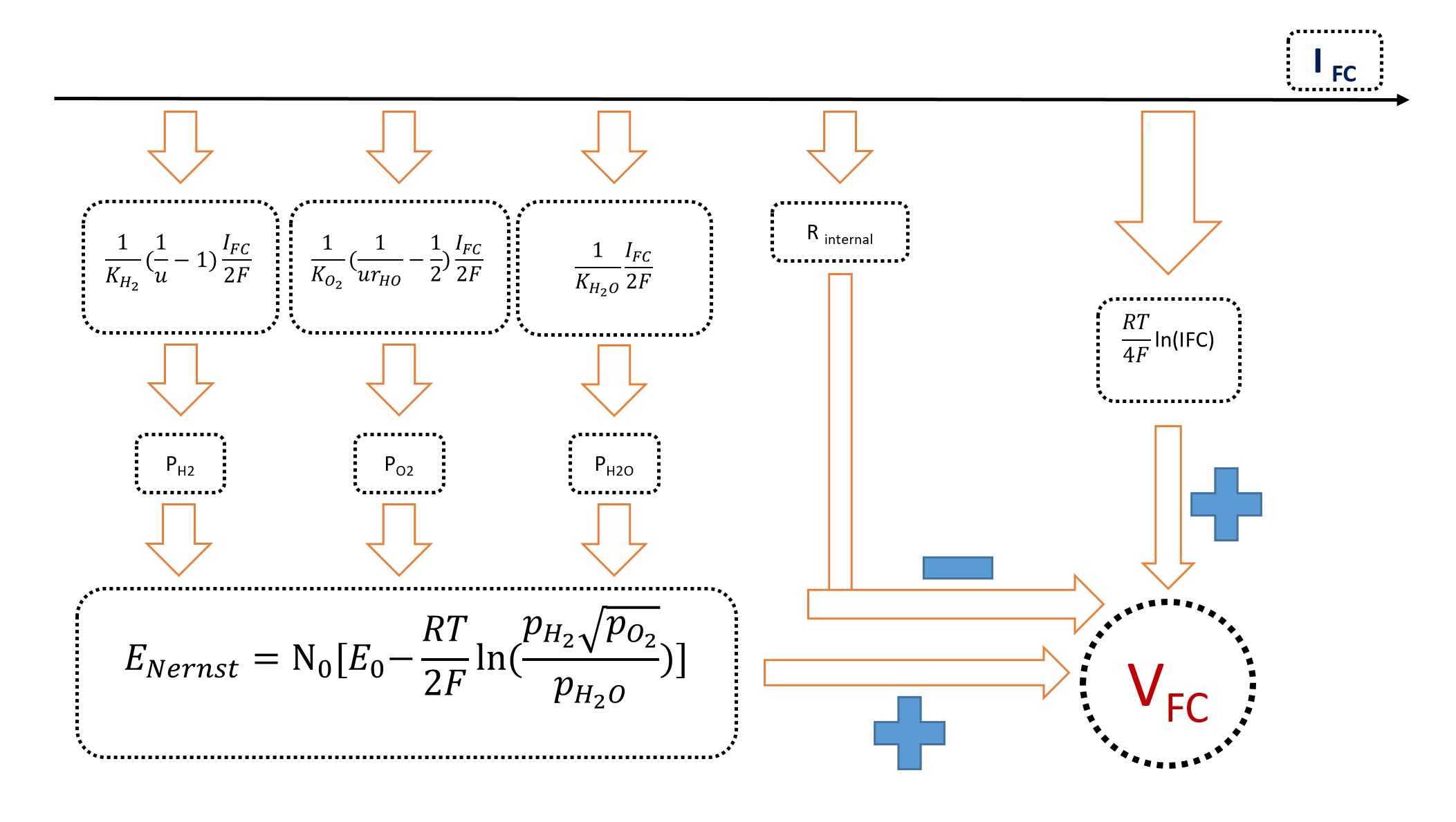
#
Fig1. Chakraborty Dynamic Model Block Diagram
#
#
# ## Nernst Voltage
# $$P_{H_2}=\frac{1}{K_{H_2}}(\frac{1}{u}-1)\frac{i}{2F}$$
# $$P_{O_2}=\frac{1}{K_{O_2}}(\frac{1}{u\times r_{HO}}-\frac{1}{2})\frac{i}{2F}$$
# $$P_{H_2O}=\frac{1}{K_{H_2O}}\frac{i}{2F}$$
# $$E_{Nernst}=N_0\times [E_0-\frac{RT}{2F}ln(\frac{P_{H_2}\times \sqrt{P_{O_2}}}{P_{H_2O}})]$$
# In[1]:
from opem.Dynamic.Chakraborty import PH2_Calc, PO2_Calc, PH2O_Calc, Enernst_Calc
# In[2]:
PH2 = PH2_Calc(KH2=0.000843, u=0.8, I=1)
PO2 = PO2_Calc(KO2=0.00252, u=0.8, rHO=1.145, I=1)
PH2O = PH2O_Calc(KH2O=0.000281, I=1)
Enernst=Enernst_Calc(E0=0.6, N0=1, T=1273, PH2=PH2, PO2=PO2, PH2O=PH2O)
Enernst
# ### FC Voltage
# $$Loss_{Ohmic}=r\times i$$
# $$Gain_{Nernst}=\frac{RT}{4F}ln(i)$$
# $$V_{Fuelcell}=E_{Nernst}+N_0[Gain_{Nernst}-Loss_{Ohmic}]$$
# In[3]:
from opem.Dynamic.Chakraborty import Vcell_Calc, Nernst_Gain_Calc, Ohmic_Loss_Calc
# In[4]:
Ohmic_Loss = Ohmic_Loss_Calc(Rint=3.28125 * 10**(-3) , I=1)
# In[5]:
Nernst_Gain = Nernst_Gain_Calc(T=1273, I=1)
# In[6]:
FC_Voltage=Vcell_Calc(Enernst=Enernst, Nernst_Gain=Nernst_Gain, Ohmic_Loss=Ohmic_Loss, N=1)
FC_Voltage
# ## Power of PEMFC
# $$P=V_{cell}\times i$$
# $$P_{Thermal}=i\times(N \times E_{th}-V_{Stack})$$
# $$E_{th}=\frac{-\Delta H}{nF}=1.23V$$
# In[7]:
from opem.Dynamic.Chakraborty import Power_Calc,Power_Thermal_Calc
Power=Power_Calc(Vcell=FC_Voltage,i=1)
Power
# In[8]:
Power_Thermal_Calc(VStack=FC_Voltage,N=1,i=1)
# ## Efficiency of PEMFC
# $$\eta=\frac{u\times V_{Fuelcell}}{N_0\times HHV}$$
# In[9]:
from opem.Dynamic.Chakraborty import Efficiency_Calc
Efficiency_Calc(Vcell=FC_Voltage,u=0.8,N=1)
# ## Linear Approximation
# Sometimes quick calculations regarding fuel cell efficiency–power-size relationships need to be made. Linear approximation is a good method to find a rough estimate of the value of polarization function at a particular point. A linear polarization curve has the following form:
# $$V_{cell}=V_0-kI$$
# where V0 is the intercept (actual open circuit voltage is always higher) and k is the slope of the curve.
#
# * Notice : Simple linear regression used for this approximation
#
#
# $$Parameter$$ |
# $$Description$$ |
# $$Unit$$ |
#
#
# $$V_0$$ |
# Intercept of the curve obtained by linear approximation |
# $$V$$ |
#
#
# $$k$$ |
# Slope of the curve obtained by linear approximation |
# $$A^{-1}$$ |
#
#
# $$P_{max}$$ |
# Maximum power obtained by linear approximation |
# $$W$$ |
#
#
# $$V_{FC}|P_{max}$$ |
# Cell voltage at maximum power obtained by linear approximation |
# $$V$$ |
#
#
#
#
#
#
#
# * Notice : These parameters are only available in HTML report
# ## Overall Parameters
#
#
# $$Parameter$$ |
# $$Description$$ |
# $$Unit$$ |
#
#
# $$\eta|P_{Max}$$ |
# Cell efficiency at maximum power |
# $$--$$ |
#
#
# $$P_{Max}$$ |
# Maximum power |
# $$W$$ |
#
#
# $$P_{Elec} $$ |
# Total electrical power |
# $$W$$ |
#
#
# $$P_{Thermal} $$ |
# Total thermal power |
# $$W$$ |
#
#
# $$V_{FC}|P_{Max}$$ |
# Cell voltage at maximum power |
# $$V$$ |
#
#
#
#
#
#
#
# * Notice : P(Thermal) & P(Elec) calculated by Simpson's Rule
#
# * Notice : These parameters are only available in HTML report
# ## Full Run
# * Run from `i`=0.1 to `i`=300 with `step`=0.1
# In[10]:
Test_Vector = {
"T": 1273,
"E0": 0.6,
"u":0.8,
"N0": 1,
"R": 3.28125 * 10**(-3),
"KH2O": 0.000281,
"KH2": 0.000843,
"KO2": 0.00252,
"rho": 1.145,
"i-start": 0.1,
"i-stop": 300,
"i-step": 0.1,
"Name": "Chakraborty_Test"}
# * Notice : "Name", new in version 0.5
# In[11]:
from opem.Dynamic.Chakraborty import Dynamic_Analysis
data=Dynamic_Analysis(InputMethod=Test_Vector,TestMode=True,PrintMode=True,ReportMode=True)
# In[12]:
data_2=Dynamic_Analysis(InputMethod=Test_Vector,TestMode=True,PrintMode=True,ReportMode=False)
# In[13]:
Dynamic_Analysis(InputMethod={},TestMode=True,PrintMode=False,ReportMode=True)
# ### Parameters
# 1. `TestMode` : Active test mode and get/return data as `dict`, (Default : `False`)
# 2. `ReportMode` : Generate reports(`.csv`,`.opem`,`.html`) and print result in console, (Default : `True`)
# 3. `PrintMode` : Control printing in console, (Default : `True`)
# 4. `Folder` : Reports folder, (Default : `os.getcwd()`)
# * Notice : "Folder" , new in version 1.4
# ## Plot
# In[14]:
import sys
get_ipython().system('{sys.executable} -m pip -qqq install matplotlib;')
import matplotlib.pyplot as plt
# In[15]:
def plot_func(x,y,x_label,y_label,color='green',legend=[],multi=False):
plt.figure()
plt.grid()
if multi==True:
for index,y_item in enumerate(y):
plt.plot(x,y_item,color=color[index])
else:
plt.plot(x,y,color=color)
plt.xlabel(x_label)
plt.ylabel(y_label)
if len(legend)!=0:
plt.legend(legend)
plt.show()
# In[16]:
plot_func(data["I"],data["P"],"I(A)","Power(W)")
# In[17]:
plot_func(data["I"],[data["V"],data["VE"]],"I(A)","V(V)",["red","blue"],legend=["Voltage-Stack","Linear-Apx"],multi=True)
# In[18]:
plot_func(data["I"],[data["Nernst Gain"],data["Ohmic Loss"]],"I(A)","V(V)",["red","blue"],legend=["Nernst Gain","Ohmic Loss"],multi=True)
# In[19]:
plot_func(data["I"],data["Ph"],"I(A)","Power-Thermal(W)","blue")
# In[20]:
plot_func(data["I"],data["EFF"],"I(A)","Efficiency","gray")
# In[21]:
plot_func(data["I"],data["PO2"],"I(A)","PO2(atm)","orange")
# In[22]:
plot_func(data["I"],data["PH2"],"I(A)","PH2(atm)","violet")
# In[23]:
plot_func(data["I"],data["PH2O"],"I(A)","PH2O(atm)","pink")
# HTML File
# OPEM File
# CSV File
# ## Parameters
# Inputs, Constants & Middle Values
# 1. User : User input
# 2. System : Simulator calculation (middle value)
#
#
# $$Parameter$$ |
# $$Description$$ |
# $$Unit$$ |
# $$Value$$ |
#
#
# $$E_0$$ |
# No load voltage |
# $$V$$ |
# $$User$$ |
#
#
# $$T$$ |
# Cell operation temperature |
# $$K$$ |
# $$User$$ |
#
#
# $$N_0$$ |
# Number of cells |
# $$−−$$ |
# $$User$$ |
#
#
# $$K_{H_2}$$ |
# Hydrogen valve constant |
# $$kmol.s^{-1}.atm^{-1}$$ |
# $$User$$ |
#
#
# $$K_{H_2O}$$ |
# Water valve constant |
# $$kmol.s^{-1}.atm^{-1}$$ |
# $$User$$ |
#
#
# $$K_{O_2}$$ |
# Oxygen valve constant |
# $$kmol.s^{-1}.atm^{-1}$$ |
# $$User$$ |
#
#
# $$R^{int}$$ |
# Fuel cell internal resistance |
# $$\Omega$$ |
# $$User$$ |
#
#
# $$r_{h-o}$$ |
# Hydrogen-Oxygen flow ratio |
# $$--$$ |
# $$User$$ |
#
#
# $$u$$ |
# Fuel utilization ratio |
# $$--$$ |
# $$User$$ |
#
#
# $$i_{start}$$ |
# Cell operating current start point |
# $$A$$ |
# $$User$$ |
#
#
# $$i_{step}$$ |
# Cell operating current step |
# $$A$$ |
# $$User$$ |
#
#
# $$i_{stop}$$ |
# Cell operating current end point |
# $$A$$ |
# $$User$$ |
#
#
# $$P_{H_2}$$ |
# Hydrogen partial pressure |
# $$atm$$ |
# $$System$$ |
#
#
# $$P_{H_2O}$$ |
# Water partial pressure |
# $$atm$$ |
# $$System$$ |
#
#
# $$P_{O_2}$$ |
# Oxygen partial pressure |
# $$atm$$ |
# $$System$$ |
#
#
# $$HHV$$ |
# Higher heating value potential |
# $$V$$ |
# $$1.482$$ |
#
#
# $$R$$ |
# Universal gas constant |
# $$J.kmol^{-1}.K^{-1}$$ |
# $$8314.47$$ |
#
#
# $$F$$ |
# Faraday’s constant |
# $$C.kmol^{-1}$$ |
# $$96484600$$ |
#
#
# $$E_{th}$$ |
# Theoretical potential |
# $$V$$ |
# $$1.23$$ |
#
#
#
#
#
# ## Reference
#
# U. Chakraborty, A New Model for Constant Fuel Utilization and Constant Fuel Flow in Fuel Cells, Appl. Sci. 9 (2019) 1066. https://doi.org/10.3390/app9061066.
#