#!/usr/bin/env python
# coding: utf-8
#
Please cite us if you use the software
# # Padulles Dynamic Model I
# ### Version 1.4
#
# ## Overview
#
# The Padulles dynamic model can predict the transient response of cell voltage, temperature of the cell, hydrogen/oxygen out flow rates and cathode and anode channel temperatures/pressures under sudden change in load current. Hence, a dynamic fuel cell simulation is developed in this model, which incorporates the dynamics of flow and pressure in the anode and cathode channels and mass/ heat transfer transient features in the fuel cell body.
#
This model is based on several assumptions :
#
#
#
# - The stack is fed with hydrogen and air
# - Cell temperature is stable at all times
# - The ratio of pressures between the interior and exterior of the electrode channels is large
# - The channels that transport gases along the electrodes have a fixed volume
# - Only source of voltage loss is ohmic polarization
# - Nernst equation can be applied too
#
#
#
#
# In this model, Nernst and fuel cell potential were modeled as a function of oxygen and hydrogen gases partial pressure that can be calculated from independent variables or constants. The partial pressure of gases is proportional to the molar flow of each gas.
#
#
#
#
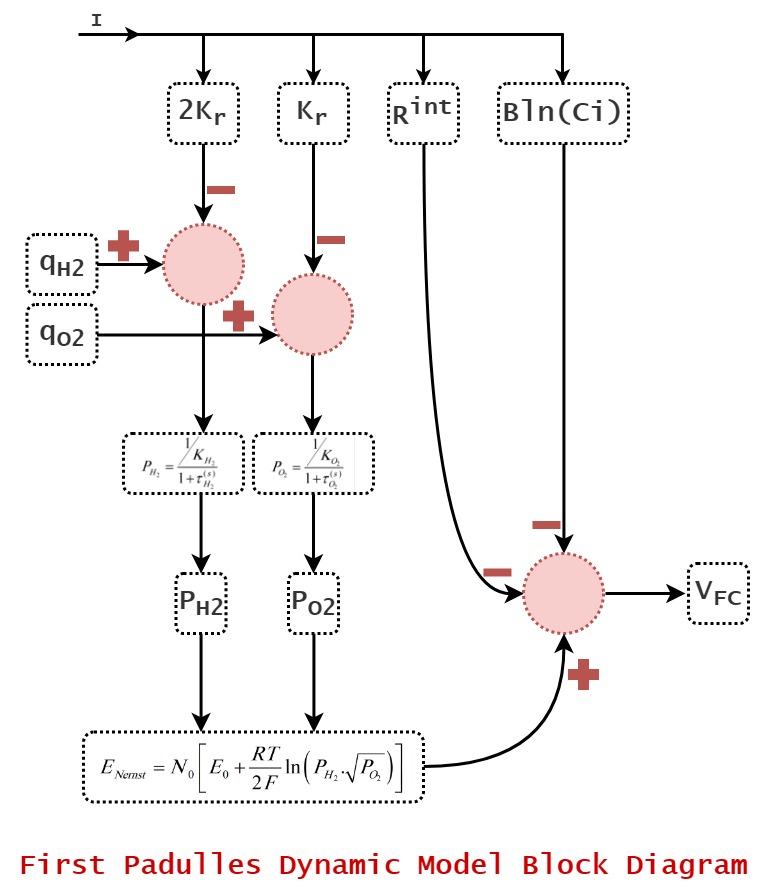
#
Fig1. Padulles-1 Dynamic Model Block Diagram
#
#
# ## Nernst Voltage
# $$E_{Nernst}=N_0\times [E_0+\frac{RT}{2F}ln(P_{H_2}\times \sqrt{P_{O_2}})]$$
# $$P_{H_2}=\frac{\frac{1}{K_{H_2}}}{1+\tau_{H_2}^{(s)}}[(q_{H_2}^{(inlet)}-(2\times K_r \times i)]$$
# $$P_{O_2}=\frac{\frac{1}{K_{O_2}}}{1+\tau_{O_2}^{(s)}}[(q_{O_2}^{(inlet)}-(K_r \times i)]$$
# $$K_r=\frac{N_0}{4F}$$
# $$q_{O_2}^{(inlet)}=\frac{q_{H_2}^{(inlet)}}{r_{h-o}}$$
# In[1]:
from opem.Dynamic.Padulles1 import Kr_Calc,qO2_Calc,PH2_Calc,PO2_Calc,Enernst_Calc
# In[2]:
Kr=Kr_Calc(N0=88)
Kr
# In[3]:
qO2=qO2_Calc(qH2=0.0004,rho=1.168)
qO2
# In[4]:
PH2=PH2_Calc(KH2=0.0000422,tH2=3.37,Kr=Kr,I=1,qH2=0.0004)
PH2
# In[5]:
PO2=PO2_Calc(KO2=0.0000211,tO2=6.74,Kr=Kr,I=1,qO2=qO2)
PO2
# In[6]:
Enernst=Enernst_Calc(E0=0.6,N0=88,T=343, PH2=PH2, PO2=PO2)
Enernst
# ### FC Voltage
# $$V_{Fuelcell}=E_{Nernst}-Bln(Ci)-(R^{int}\times i)$$
# In[7]:
from opem.Dynamic.Padulles1 import Vcell_Calc
FC_Voltage=Vcell_Calc(Enernst=Enernst, B=0.04777,C=0.0136,I=1,Rint=0.00303)
FC_Voltage
# ## Power of PEMFC
# $$P=V_{cell}\times i$$
# $$P_{Thermal}=i\times(N \times E_{th}-V_{Stack})$$
# $$E_{th}=\frac{-\Delta H}{nF}=1.23V$$
# In[8]:
from opem.Dynamic.Padulles1 import Power_Calc,Power_Thermal_Calc
Power=Power_Calc(Vcell=FC_Voltage,i=2)
Power
# In[9]:
Power_Thermal_Calc(VStack=FC_Voltage,N=88,i=2)
# ## Efficiency of PEMFC
# $$\eta=\frac{\mu_F\times V_{Fuelcell}}{N_0\times HHV}$$
# In[10]:
from opem.Dynamic.Padulles1 import Efficiency_Calc
Efficiency_Calc(Vcell=FC_Voltage,N=88)
# ## Linear Approximation
# Sometimes quick calculations regarding fuel cell efficiency–power-size relationships need to be made. Linear approximation is a good method to find a rough estimate of the value of polarization function at a particular point. A linear polarization curve has the following form:
# $$V_{cell}=V_0-kI$$
# where V0 is the intercept (actual open circuit voltage is always higher) and k is the slope of the curve.
#
# * Notice : Simple linear regression used for this approximation
#
#
# $$Parameter$$ |
# $$Description$$ |
# $$Unit$$ |
#
#
# $$V_0$$ |
# Intercept of the curve obtained by linear approximation |
# $$V$$ |
#
#
# $$k$$ |
# Slope of the curve obtained by linear approximation |
# $$A^{-1}$$ |
#
#
# $$P_{max}$$ |
# Maximum power obtained by linear approximation |
# $$W$$ |
#
#
# $$V_{FC}|P_{max}$$ |
# Cell voltage at maximum power obtained by linear approximation |
# $$V$$ |
#
#
#
#
#
#
#
# * Notice : These parameters are only available in HTML report
# ## Overall Parameters
#
#
# $$Parameter$$ |
# $$Description$$ |
# $$Unit$$ |
#
#
# $$\eta|P_{Max}$$ |
# Cell efficiency at maximum power |
# $$--$$ |
#
#
# $$P_{Max}$$ |
# Maximum power |
# $$W$$ |
#
#
# $$P_{Elec} $$ |
# Total electrical power |
# $$W$$ |
#
#
# $$P_{Thermal} $$ |
# Total thermal power |
# $$W$$ |
#
#
# $$V_{FC}|P_{Max}$$ |
# Cell voltage at maximum power |
# $$V$$ |
#
#
#
#
#
#
#
# * Notice : P(Thermal) & P(Elec) calculated by Simpson's Rule
#
# * Notice : These parameters are only available in HTML report
# ## Full Run
# * Run from `i`=0 to `i`=100 with `step`=0.1
# In[11]:
Test_Vector = {
"T": 343,
"E0": 0.6,
"N0": 88,
"KO2": 0.0000211,
"KH2": 0.0000422,
"tH2": 3.37,
"tO2": 6.74,
"B": 0.04777,
"C": 0.0136,
"Rint": 0.00303,
"rho": 1.168,
"qH2": 0.0004,
"i-start": 0,
"i-stop": 100,
"i-step": 0.1,
"Name": "PadullesI_Test"}
# * Notice : "Name", new in version 0.5
# In[12]:
from opem.Dynamic.Padulles1 import Dynamic_Analysis
data=Dynamic_Analysis(InputMethod=Test_Vector,TestMode=True,PrintMode=True,ReportMode=True)
# * Notice : "Status", "V0", "K" and "EFF" , new in version 0.8
# In[13]:
data_2=Dynamic_Analysis(InputMethod=Test_Vector,TestMode=True,PrintMode=True,ReportMode=False)
# In[14]:
Dynamic_Analysis(InputMethod={},TestMode=True,PrintMode=False,ReportMode=True)
# ### Parameters
# 1. `TestMode` : Active test mode and get/return data as `dict`, (Default : `False`)
# 2. `ReportMode` : Generate reports(`.csv`,`.opem`,`.html`) and print result in console, (Default : `True`)
# 3. `PrintMode` : Control printing in console, (Default : `True`)
# 4. `Folder` : Reports folder, (Default : `os.getcwd()`)
# * Notice : "PrintMode" & "ReportMode" , new in version 0.5
# * Notice : "Folder" , new in version 1.4
# ## Plot
# In[15]:
import sys
get_ipython().system('{sys.executable} -m pip -qqq install matplotlib;')
import matplotlib.pyplot as plt
# In[16]:
def plot_func(x,y,x_label,y_label,color='green',legend=[],multi=False):
plt.figure()
plt.grid()
if multi==True:
for index,y_item in enumerate(y):
plt.plot(x,y_item,color=color[index])
else:
plt.plot(x,y,color=color)
plt.xlabel(x_label)
plt.ylabel(y_label)
if len(legend)!=0:
plt.legend(legend)
plt.show()
# In[17]:
plot_func(data["I"],data["P"],"I(A)","Power(W)")
# In[18]:
plot_func(data["I"],[data["V"],data["VE"]],"I(A)","V(V)",["red","blue"],legend=["Voltage-Stack","Linear-Apx"],multi=True)
# In[19]:
plot_func(data["I"],data["Ph"],"I(A)","Power-Thermal(W)","blue")
# In[20]:
plot_func(data["I"],data["EFF"],"I(A)","Efficiency","gray")
# In[21]:
plot_func(data["I"],data["PO2"],"I(A)","PO2(atm)","orange")
# In[22]:
plot_func(data["I"],data["PH2"],"I(A)","PH2(atm)","violet")
# HTML File
# OPEM File
# CSV File
# ## Parameters
# Inputs, Constants & Middle Values
# 1. User : User input
# 2. System : Simulator calculation (middle value)
#
#
# $$Parameter$$ |
# $$Description$$ |
# $$Unit$$ |
# $$Value$$ |
#
#
# $$E_0$$ |
# No load voltage |
# $$V$$ |
# $$User$$ |
#
#
# $$T$$ |
# Fuel cell temperature |
# $$K$$ |
# $$User$$ |
#
#
# $$N_0$$ |
# Number of cells |
# $$--$$ |
# $$User$$ |
#
#
# $$K_{H_2}$$ |
# Hydrogen valve constant |
# $$kmol.s^{-1}.atm^{-1}$$ |
# $$User$$ |
#
#
# $$K_{O_2}$$ |
# Oxygen valve constant |
# $$kmol.s^{-1}.atm^{-1}$$ |
# $$User$$ |
#
#
# $$\tau_{H_2}^{(s)}$$ |
# Hydrogen time constant |
# $$s$$ |
# $$User$$ |
#
#
# $$\tau_{O_2}^{(s)}$$ |
# Oxygen time constant |
# $$s$$ |
# $$User$$ |
#
#
# $$B$$ |
# Activation voltage constant |
# $$V$$ |
# $$User$$ |
#
#
# $$C$$ |
# Activation constant parameter |
# $$A^{-1}$$ |
# $$User$$ |
#
#
# $$R^{int}$$ |
# Fuel cell internal resistance |
# $$\Omega$$ |
# $$User$$ |
#
#
# $$r_{h-o}$$ |
# Hydrogen-Oxygen flow ratio |
# $$--$$ |
# $$User$$ |
#
#
# $$q_{H_2}^{(inlet)}$$ |
# Molar flow of hydrogen |
# $$kmol.s^{-1}$$ |
# $$User$$ |
#
#
# $$i_{start}$$ |
# Cell operating current start point |
# $$A$$ |
# $$User$$ |
#
#
# $$i_{step}$$ |
# Cell operating current step |
# $$A$$ |
# $$User$$ |
#
#
# $$i_{stop}$$ |
# Cell operating current end point |
# $$A$$ |
# $$User$$ |
#
#
# $$P_{H_2}$$ |
# Hydrogen partial pressure |
# $$atm$$ |
# $$System$$ |
#
#
# $$P_{O_2}$$ |
# Oxygen partial pressure |
# $$atm$$ |
# $$System$$ |
#
#
# $$K_r$$ |
# Modeling constant |
# $$kmol.s^{-1}.A^{-1}$$ |
# $$System$$ |
#
#
# $$q_{O_2}^{(inlet)}$$ |
# Molar flow of oxygen |
# $$kmol.s^{-1}$$ |
# $$System$$ |
#
#
# $$\mu_F$$ |
# The fuel utilization |
# $$--$$ |
# $$0.95$$ |
#
#
# $$HHV$$ |
# Higher heating value potential |
# $$V$$ |
# $$1.482$$ |
#
#
# $$R$$ |
# Universal gas constant |
# $$J.kmol^{-1}.K^{-1}$$ |
# $$8314.47$$ |
#
#
# $$F$$ |
# Faraday’s constant |
# $$C.kmol^{-1}$$ |
# $$96484600$$ |
#
#
# $$E_{th}$$ |
# Theoretical potential |
# $$V$$ |
# $$1.23$$ |
#
#
#
#
#
# ## Reference
#
# J. Padulles, G.W. Ault, J.R. McDonald. 2000. "An integrated SOFC plant dynamic model for power systems
# simulation." Journal of Power Sources (Elsevier) 86 (1-2): 495-500. doi:10.1016/S0378-7753(99)00430-9.
#