#!/usr/bin/env python # coding: utf-8 # 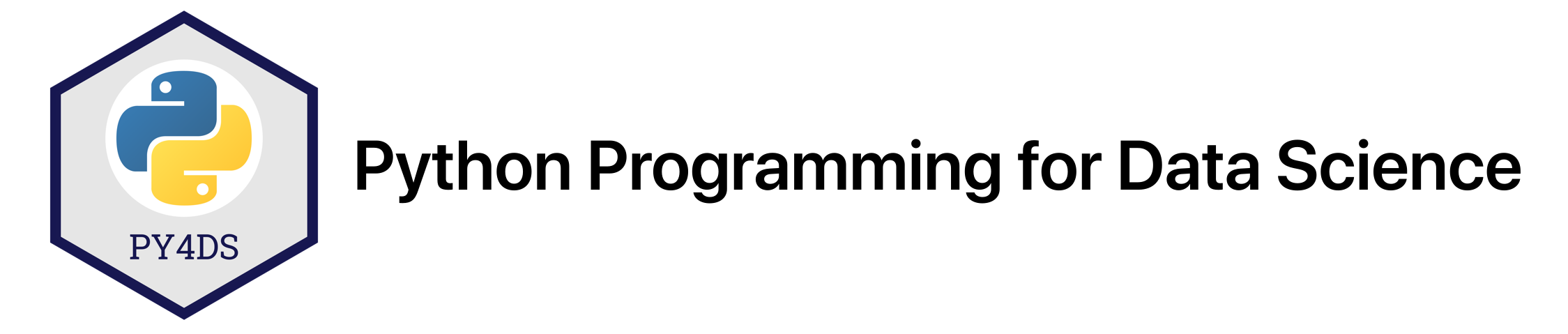 # # NumPy # # **Tomas Beuzen, September 2020** # These exercises complement [Chapter 5](../chapters/chapter5-numpy.ipynb) and [Chapter 6](../chapters/chapter6-numpy-addendum.ipynb). # ## Exercises # ### 1. # Import numpy under the alias `np`. # In[1]: # Your answer here. # ### 2. # Create the following arrays: # # 1. Create an array of 5 zeros. # 2. Create an array of 10 ones. # 3. Create an array of 5 3.141s. # 4. Create an array of the integers 1 to 20. # 5. Create a 5 x 5 matrix of ones with a dtype `int`. # In[2]: # Your answer here. # ### 3. # Use numpy to: # 1. Create an 3D matrix of 3 x 3 x 3 full of random numbers drawn from a standard normal distribution (hint: `np.random.randn()`) # 2. Reshape the above array into shape (27,) # In[3]: # Your answer here. # ### 4. # Create an array of 20 linearly spaced numbers between 1 and 10. # In[4]: # Your answer here. # ### 5. # Run the following code to create an array of shape 4 x 4 and then use indexing to produce the outputs shown below. # In[5]: import numpy as np a = np.arange(1, 26).reshape(5, -1) # ```python # 20 # ``` # In[6]: # Your answer here. # ```python # array([[ 9, 10], # [14, 15], # [19, 20], # [24, 25]]) # ``` # In[7]: # Your answer here. # ```python # array([ 6, 7, 8, 9, 10]) # ``` # In[8]: # Your answer here. # ```python # array([[11, 12, 13, 14, 15], # [16, 17, 18, 19, 20]]) # ``` # In[9]: # Your answer here. # ```python # array([[ 8, 9], # [13, 14]]) # ``` # In[10]: # Your answer here. # ### 6. # Calculate the sum of all the numbers in `a`. # In[11]: # Your answer here. # ### 7. # Calculate the sum of each row in `a`. # In[12]: # Your answer here. # ### 8. # Extract all values of `a` greater than the mean of `a` (hint: use a boolean mask). # In[13]: # Your answer here. # ### 9. # Find the location of the minimum value in the following array `b`: # In[14]: np.random.seed(123) b = np.random.randn(10) b # In[15]: # Your answer here. # ### 10. # Find the location of the maximum value in the following 2D array `c` (hint: there are many ways to do this question, but a quick search on stackoverflow.com will typically help you find the optimum solution for a problem, for example see [post](https://stackoverflow.com/questions/3584243/get-the-position-of-the-biggest-item-in-a-multi-dimensional-numpy-array)): # In[16]: np.random.seed(123) c = np.random.randn(3, 2) c # In[17]: # Your answer here. #