#!/usr/bin/env python # coding: utf-8 # 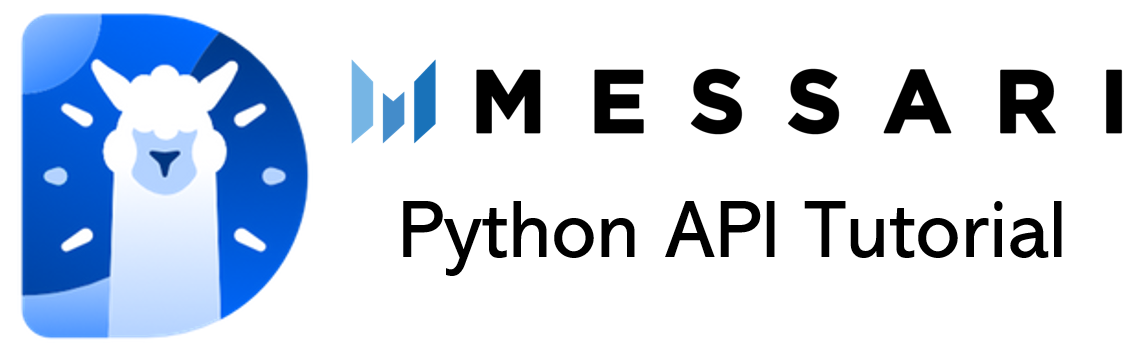 # # DeFi Llama Python API Tutorial # This tutorial aims to be a quick guide to get you started using the DeFi Llama API integrated into messari's python library. # In[2]: from messari.defillama import DeFiLlama dl = DeFiLlama() # ## API Structure # The Messari Python client contains a number of functions that wrap all of DeFi Llama's API endpoints. These include: # # * get_protocol_tvl_timeseries # * get_global_tvl_timeseries # * get_chain_tvl_timeseries # * get_current_tvl # * get_protocols # # Below are a few examples to showcase the functionality and types of data each function generates. # ## Get protocol tvl timeseries # This function returns a timeseries of a protocol's TVL broken down by token amounts as a pandas DataFrame. The DataFrame uses a multiindex to group relevant data together following a convenient **df[protocol][chain][asset]** pattern. For example, the following code returns Aave's aggregate TVL and token amounts across all chains: # In[3]: protocols = ['aave'] protocol_tvls = dl.get_protocol_tvl_timeseries(protocols, start_date="2021-10-01", end_date="2021-10-10") protocol_tvls['aave']['all'].head() # We could narrow down our search by passing other supported chains as follows: # In[6]: protocol_tvls['aave']['Ethereum'].head() # To look at a protocol's aggregate TVL across all tokens of one specific chain, pass 'totalLiquidityUSD' as the asset index. For example, if we wanted to know Aave's total TVL in Ethereum, we would run: # In[7]: protocol_tvls['aave']['Ethereum']['totalLiquidityUSD'] # ## Get global tvl timeseries # This function returns a timeseries of aggregate TVL across all supported protocols in DeFi Llama as a pandas DataFrame # In[3]: global_tvl = dl.get_global_tvl_timeseries(start_date="2021-10-01", end_date="2021-10-10") global_tvl # ## Get chain tvl timeseries # This function retrives timeseries TVL for a given chain or list of chains as a pandas DataFrame # In[4]: chains = ["Avalanche", "Harmony", "Polygon"] chain_tvls = dl.get_chain_tvl_timeseries(chains, start_date="2021-10-01", end_date="2021-10-10") chain_tvls # ## Get current tvl # The function retrives the current protocol tvl as a pandas DataFrame for an asset or list of assets # In[8]: protocols = ["uniswap", "curve", "aave"] current_tvl = dl.get_current_tvl(protocols) current_tvl # ## Get protocols # The function returns basic information on all DeFi Llama listed protocols. # In[10]: protocols = dl.get_protocols() protocols # In[ ]: