#!/usr/bin/env python
# coding: utf-8
#
# All the IPython Notebooks in **Python Introduction** lecture series by **[Dr. Milaan Parmar](https://www.linkedin.com/in/milaanparmar/)** are available @ **[GitHub](https://github.com/milaan9/01_Python_Introduction)**
#
# # Learn Python Programming
#
#
#
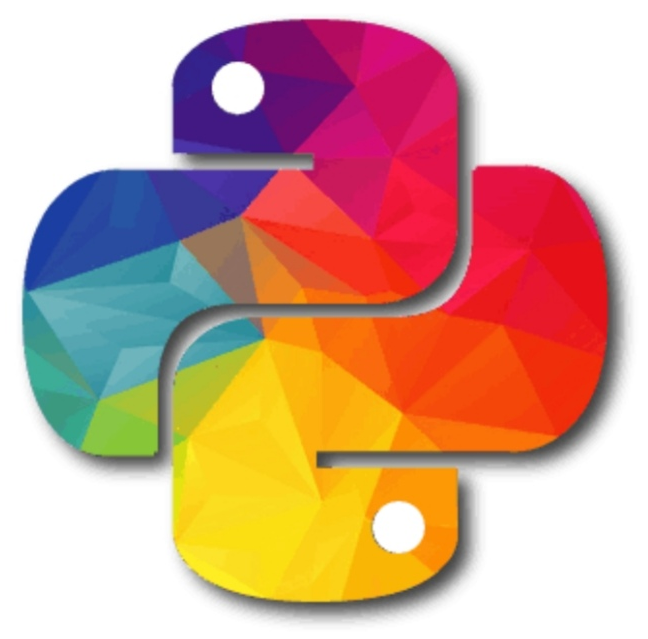
#
#
# Python is a **general-purpose, mordern, dynamic, robust, high level** and **interpreted** programming language. It is used in web development, data science, creating software prototypes, and so on. Fortunately for beginners, Python has simple easy-to-use syntax. This makes Python an excellent language to learn to program for beginners.
# ## [Python Introduction](https://github.com/milaan9/01_Python_Introduction)
#
# ✅ **[What is Programming?](https://github.com/milaan9/01_Python_Introduction/blob/main/0000_What_is_Programming.ipynb)**
#
# ✅ **[Introduction to Python](https://github.com/milaan9/01_Python_Introduction/blob/main/000_Intro_to_Python.ipynb)**
#
# ✅ **[Python Programming](https://github.com/milaan9/01_Python_Introduction/blob/main/001_Python_Programming.ipynb)**
#
# ✅ **[Getting Started](https://github.com/milaan9/01_Python_Introduction/blob/main/002_How_to_install_Python.ipynb)**
#
# ✅ **[Jupyter Keyboard Shortcuts Practice](https://github.com/milaan9/01_Python_Introduction/blob/main/003_Jupyter_Keyboard_Shortcuts_Practice.ipynb)**
#
# ✅ **[Hello World](https://github.com/milaan9/01_Python_Introduction/blob/main/004_Hello_World.ipynb)**
#
# ✅ **[Keywords and Identifiers](https://github.com/milaan9/01_Python_Introduction/blob/main/005_Python_Keywords_and_Identifiers.ipynb)**
#
# ✅ **[Statements, Indentation & Comments](https://github.com/milaan9/01_Python_Introduction/blob/main/006_Python_Statement_Indentation_Comments.ipynb)**
#
# ✅ **[Python Variables & Constants](https://github.com/milaan9/01_Python_Introduction/blob/main/007_Python_Variables_%26_Constants.ipynb)**
#
# ✅ **[Python Literals](https://github.com/milaan9/01_Python_Introduction/blob/main/008_Python_Literals.ipynb)**
#
# ✅ **[Python Datatypes](https://github.com/milaan9/01_Python_Introduction/blob/main/009_Python_Data_Types.ipynb)**
#
# ✅ **[Python Type Conversion](https://github.com/milaan9/01_Python_Introduction/blob/main/010_Python_Type_Conversion.ipynb)**
#
# ✅ **[Python I/O and import](https://github.com/milaan9/01_Python_Introduction/blob/main/011_Python_Input_Output_Import.ipynb)**
#
# ✅ **[Python Operators](https://github.com/milaan9/01_Python_Introduction/blob/main/012_Python_Operators.ipynb)**
#
# ✅ **[Python Namespace & Scope](https://github.com/milaan9/01_Python_Introduction/blob/main/013_Python_Namespace_and_Scope.ipynb)**
#
# ✅ **[Python Keyword List](https://github.com/milaan9/01_Python_Introduction/blob/main/Python_Keywords_List.ipynb)**
# ## [Python Data Types](https://github.com/milaan9/02_Python_Datatypes)
#
# ✅ **[Python Numbers](https://github.com/milaan9/02_Python_Datatypes/blob/main/001_Python_Numbers.ipynb)**
#
# ✅ **[Python String](https://github.com/milaan9/02_Python_Datatypes/blob/main/002_Python_String.ipynb)**
#
# ✅ **[Python List](https://github.com/milaan9/02_Python_Datatypes/blob/main/003_Python_List.ipynb)**
#
# ✅ **[Python Tuple](https://github.com/milaan9/02_Python_Datatypes/blob/main/004_Python_Tuple.ipynb)**
#
# ✅ **[Python Dictionary](https://github.com/milaan9/02_Python_Datatypes/blob/main/005_Python_Dictionary.ipynb)**
#
# ✅ **[Python Sets](https://github.com/milaan9/02_Python_Datatypes/blob/main/006_Python_Sets.ipynb)**
# ## [Python Flow Control](https://github.com/milaan9/03_Python_Flow_Control)
#
# ✅ **[Python Flow Control Statement](https://github.com/milaan9/03_Python_Flow_Control/blob/main/000_Python_Flow_Control_statement%20.ipynb)**
#
# ✅ **[Python if statement](https://github.com/milaan9/03_Python_Flow_Control/blob/main/001_Python_if_statement.ipynb)**
#
# ✅ **[Python if-else statement](https://github.com/milaan9/03_Python_Flow_Control/blob/main/002_Python_if_else_statement.ipynb)**
#
# ✅ **[Python if-elif-else statement](https://github.com/milaan9/03_Python_Flow_Control/blob/main/003_Python_if_elif_else_statement%20.ipynb)**
#
# ✅ **[Python Nested if statement](https://github.com/milaan9/03_Python_Flow_Control/blob/main/004_Python_Nested_if_statement.ipynb)**
#
# ✅ **[Python for loop](https://github.com/milaan9/03_Python_Flow_Control/blob/main/005_Python_for_Loop.ipynb)**
#
# ✅ **[Python while loop](https://github.com/milaan9/03_Python_Flow_Control/blob/main/006_Python_while_Loop.ipynb)**
#
# ✅ **[Python break, continue & pass statements](https://github.com/milaan9/03_Python_Flow_Control/blob/main/007_Python_break_continue_pass_statements.ipynb)**
# ## [Python Functions](https://github.com/milaan9/04_Python_Functions)
#
# ✅ **[Python Functions](https://github.com/milaan9/04_Python_Functions/blob/main/001_Python_Functions.ipynb)**
#
# ✅ **[Global, Local & Nonlocal](https://github.com/milaan9/04_Python_Functions/blob/main/002_Python_Function_Global_Local_Nonlocal.ipynb)**
#
# ✅ **[Global keyword](https://github.com/milaan9/04_Python_Functions/blob/main/003_Python_Function_global_Keywords.ipynb)**
#
# ✅ **[Function Argument](https://github.com/milaan9/04_Python_Functions/blob/main/004_Python_Function_Arguments.ipynb)**
#
# ✅ **[Function Recursion](https://github.com/milaan9/04_Python_Functions/blob/main/005_Python_Function_Recursion.ipynb)**
#
# ✅ **[Function Anonymous](https://github.com/milaan9/04_Python_Functions/blob/main/006_Python_Function_Anonymous.ipynb)**
#
# ✅ **[Function Module](https://github.com/milaan9/04_Python_Functions/blob/main/007_Python_Function_Module.ipynb)**
#
# ✅ **[Function random Module](https://github.com/milaan9/04_Python_Functions/blob/main/008_Python_Function_random_Module.ipynb)**
#
# ✅ **[Function math Module](https://github.com/milaan9/04_Python_Functions/blob/main/009_Python_Function_math_Module.ipynb.ipynb)**
#
# ✅ **[Function Package](https://github.com/milaan9/04_Python_Functions/blob/main/010_Python_Function_Package.ipynb)**
#
# ✅ **[Python Docstrings](https://github.com/milaan9/04_Python_Functions/blob/main/Python_Docstrings.ipynb)**
#
# ✅ **[User-defined Function](https://github.com/milaan9/04_Python_Functions/blob/main/Python_User_defined_Functions.ipynb)**
# ## [Python Files](https://github.com/milaan9/05_Python_Files)
#
# ✅ **[Python File I/O](https://github.com/milaan9/05_Python_Files/blob/main/001_Python_File_Input_Output.ipynb)**
#
# ✅ **[Python File Directory](https://github.com/milaan9/05_Python_Files/blob/main/002_Python_File_Directory.ipynb)**
#
# ✅ **[Python File Exception](https://github.com/milaan9/05_Python_Files/blob/main/003_Python_File_Exception.ipynb)**
#
# ✅ **[Python Exceptions Handeling](https://github.com/milaan9/05_Python_Files/blob/main/004_Python_Exceptions_Handling.ipynb)**
#
# ✅ **[Python User-defined Exceptions](https://github.com/milaan9/05_Python_Files/blob/main/005_Python_User_defined_Exceptions.ipynb)**
# ## [Python Object Class](https://github.com/milaan9/06_Python_Object_Class)
#
# ✅ **[Python OOPs Concepts](https://github.com/milaan9/06_Python_Object_Class/blob/main/001_Python_OOPs_Concepts.ipynb)**
#
# ✅ **[Python Classes & Objects](https://github.com/milaan9/06_Python_Object_Class/blob/main/002_Python_Classes_and_Objects.ipynb)**
#
# ✅ **[Python Inheritance](https://github.com/milaan9/06_Python_Object_Class/blob/main/003_Python_Inheritance.ipynb)**
#
# ✅ **[Python Operator Overloading](https://github.com/milaan9/06_Python_Object_Class/blob/main/004_Python_Operator_Overloading.ipynb)**
# ## [Python Advanced Topics](https://github.com/milaan9/07_Python_Advanced_Topics)
#
# ✅ **[Python Iterator](https://github.com/milaan9/07_Python_Advanced_Topics/blob/main/001_Python_Iterators.ipynb)**
#
# ✅ **[Python Generator](https://github.com/milaan9/07_Python_Advanced_Topics/blob/main/002_Python_Generators.ipynb)**
#
# ✅ **[Python Closure](https://github.com/milaan9/07_Python_Advanced_Topics/blob/main/003_Python_Closure.ipynb)**
#
# ✅ **[Python Decorators](https://github.com/milaan9/07_Python_Advanced_Topics/blob/main/004_Python_Decorators.ipynb)**
#
# ✅ **[Python Property](https://github.com/milaan9/07_Python_Advanced_Topics/blob/main/005_Python_Property.ipynb)**
#
# ✅ **[Python RegEx](https://github.com/milaan9/07_Python_Advanced_Topics/blob/main/006_Python_RegEx.ipynb)**
# ## [Python Date & Time Module](https://github.com/milaan9/08_Python_Date_Time_Module)
#
# ✅ **[Python datetime Module](https://github.com/milaan9/08_Python_Date_Time_Module/blob/main/001_Python_datetime_Module.ipynb)**
#
# ✅ **[Python datetime.strftime()](https://github.com/milaan9/08_Python_Date_Time_Module/blob/main/002_Python_strftime%28%29.ipynb)**
#
# ✅ **[Python datetime.strptime()](https://github.com/milaan9/08_Python_Date_Time_Module/blob/main/003_Python_strptime%28%29.ipynb)**
#
# ✅ **[Current date & time](https://github.com/milaan9/08_Python_Date_Time_Module/blob/main/004_Python_current_date_and_time.ipynb)**
#
# ✅ **[Get current time](https://github.com/milaan9/08_Python_Date_Time_Module/blob/main/005_Python_get_current_time.ipynb)**
#
# ✅ **[Timestamp to datetime](https://github.com/milaan9/08_Python_Date_Time_Module/blob/main/006_Python_timestamp_to_datetime.ipynb)**
#
# ✅ **[Python time Module](https://github.com/milaan9/08_Python_Date_Time_Module/blob/main/007_Python_time_Module.ipynb)**
#
# ✅ **[Python time.sleep()](https://github.com/milaan9/08_Python_Date_Time_Module/blob/main/008_Python_sleep%28%29.ipynb)**
# ## [Python Numpy Module](https://github.com/milaan9/09_Python_NumPy_Module)
#
# ✅ **[Python Numpy](https://github.com/milaan9/09_Python_NumPy_Module/blob/main/001_Python_NumPy.ipynb)**
#
# ✅ **[Python Numpy Array](https://github.com/milaan9/09_Python_NumPy_Module/blob/main/002_Python_NumPy_Array.ipynb)**
# ## [Python Pandas Module](https://github.com/milaan9/10_Python_Pandas_Module)
#
# ✅ **[Python Pandas Dataframe](https://github.com/milaan9/10_Python_Pandas_Module/blob/main/001_Python_Pandas_DataFrame.ipynb)**
#
# ✅ **[Python Pandas Exercise 1](https://github.com/milaan9/10_Python_Pandas_Module/blob/main/002_Python_Pandas_Exercise_1.ipynb)**
#
# ✅ **[Python Pandas Exercise 2](https://github.com/milaan9/10_Python_Pandas_Module/blob/main/003_Python_Pandas_Exercise_2.ipynb)**
# ## [Python Matplotlib Module](https://github.com/milaan9/11_Python_Matplotlib_Module)
#
# ✅ **[Python Matplotlib pyplot]()**
#
# ✅ **[Python Matplotlib Exercise 1](https://github.com/milaan9/11_Python_Matplotlib_Module/blob/main/002_Python_Matplotlib_Exercise_1.ipynb)**
#
# ✅ **[Python Matplotlib Exercise 2](https://github.com/milaan9/11_Python_Matplotlib_Module/blob/main/003_Python_Matplotlib_Exercise_2.ipynb)**
# ## About Python Programming
#
# * **Free and open-source** - You can freely use and distribute Python, even for commercial use.
# * **Easy to learn** - Python has a very simple and elegant syntax. It's much easier to read and write Python programs compared to other languages like C++, Java, C#.
# * **Portable** - You can move Python programs from one platform to another, and run it without any changes.
# ## Why Learn Python?
#
# * Python is easy to learn. Its syntax is easy and code is very readable.
# * Python has a lot of applications. It's used for developing web applications, data science, rapid application development, and so on.
# * Python allows you to write programs in fewer lines of code than most of the programming languages.
# * The popularity of Python is growing rapidly. Now it's one of the most popular programming languages.
# ## How to learn Python?
#
# * **Python classes** - We provide step by step Python tutorials, examples, and references. **[Get started with Python]()**.
# * **Official Python tutorial** - Might be hard to follow and understand for beginners. Visit the official **[Python tutorial](https://docs.python.org/3/tutorial/)**.
# * **Write a lot of Python code** - The only way you can learn programming is by writing a lot of code.
# ## Python Resources
#
# * **[Python Examples](https://github.com/milaan9/90_Python_Examples)**
# * **[Python References](https://github.com/milaan9/04_Python_Functions/tree/main/002_Python_Functions_Built_in)**
# * **[Python Online Compiler 1](https://www.w3schools.com/python/trypython.asp?filename=demo_compiler)**
# * **[Python Online Compiler 2](https://www.tutorialspoint.com/execute_python_online.php)**
# * **[Python Guide](https://github.com/milaan9/01_Python_Introduction/blob/main/001_Python_Programming.ipynb)**
#