#!/usr/bin/env python
# coding: utf-8
# # Introduction
#
# QA plots for the INTT detector
# In[1]:
# imports to write dynamic markdown contents
import os
from IPython.display import display, Markdown, Latex
from IPython.display import HTML
# In[2]:
# turn off/on code for the result HTML page
display(Markdown('*For the result HTML page:* '))
HTML('''
''')
# In[3]:
import os.path
# readme file of the macros, available if run under JenkinsCI
# https://github.com/sPHENIX-Collaboration/utilities/blob/master/jenkins/built-test/test-tracking-qa.sh
macro_markdown = 'Fun4All-macros-README.md'
if os.path.isfile(macro_markdown) :
with open(macro_markdown, 'r') as file:
display(Markdown(file.read()))
# ## `pyROOT` env check
# In[4]:
import ROOT
OFFLINE_MAIN = os.getenv("OFFLINE_MAIN")
if OFFLINE_MAIN is not None:
display(Markdown(f"via sPHENIX software distribution at `{OFFLINE_MAIN}`"))
# ## Plotting source code
# In[5]:
import subprocess
try:
git_url = \
subprocess.run(['git','remote','get-url','origin'], stdout=subprocess.PIPE)\
.stdout.decode('utf-8').strip()\
.replace('git@github.com:','https://github.com/')
display(Markdown(f"View the source code repository at {git_url}"))
except: # catch *all* exceptions
# well do nothing
pass
# ## JenkinsCI information (if available)
# In[6]:
display(Markdown('Some further details about the QA run, if executed under the Jenkins CI:'))
checkrun_repo_commit = os.getenv("checkrun_repo_commit")
if checkrun_repo_commit is not None:
display(Markdown(f"* The commit being checked is {checkrun_repo_commit}"))
ghprbPullLink = os.getenv("ghprbPullLink")
if ghprbPullLink is not None:
display(Markdown(f"* Link to the pull request: {ghprbPullLink}"))
BUILD_URL = os.getenv("BUILD_URL")
if BUILD_URL is not None:
display(Markdown(f"* Link to the build: {BUILD_URL}"))
git_url_macros = os.getenv("git_url_macros")
sha_macros = os.getenv("sha_macros")
if git_url_macros is not None:
display(Markdown(f"* Git repo for macros: {git_url_macros} , which merges `{sha_macros}` and the QA tracking branch"))
RUN_ARTIFACTS_DISPLAY_URL = os.getenv("RUN_ARTIFACTS_DISPLAY_URL")
if RUN_ARTIFACTS_DISPLAY_URL is not None:
display(Markdown(f"* Download the QA ROOT files: {RUN_ARTIFACTS_DISPLAY_URL}"))
JENKINS_URL = os.getenv("JENKINS_URL")
if JENKINS_URL is not None:
display(Markdown(f"Automatically generated by [sPHENIX Jenkins continuous integration]({JENKINS_URL}) [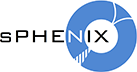](https://www.sphenix.bnl.gov/web/) [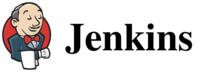](https://jenkins.io/)"))
#
# # Initialization
# In[7]:
get_ipython().run_cell_magic('cpp', '-d', '\n#include "QA_Draw_Utility.C"\n\n#include \n\n#include \n#include \n#include \n#include \n#include \n#include \n')
# In[8]:
get_ipython().run_cell_magic('cpp', '', '\nSetsPhenixStyle();\nTVirtualFitter::SetDefaultFitter("Minuit2");\n\n// test sPHENIX lib load\n// gSystem->Load("libg4eval.so");\n\n// test libs\n// gSystem->ListLibraries();\n')
# In[9]:
get_ipython().run_line_magic('jsroot', 'on')
# ## Inputs and file checks
# In[10]:
qa_file_name_new = os.getenv("qa_file_name_new")
if qa_file_name_new is None:
qa_file_name_new = "G4sPHENIX_test-tracking-low-occupancy-qa_Event100_Sum10_qa.root"
display(Markdown(f"`qa_file_name_new` env not set. use the default `qa_file_name_new={qa_file_name_new}`"))
qa_file_name_ref = os.getenv("qa_file_name_ref")
if qa_file_name_ref is None:
qa_file_name_ref = "reference/G4sPHENIX_test-tracking-low-occupancy-qa_Event100_Sum10_qa.root"
display(Markdown(f"`qa_file_name_ref` env not set. use the default `qa_file_name_ref={qa_file_name_ref}`"))
elif qa_file_name_ref == 'None':
qa_file_name_ref = None
display(Markdown(f"`qa_file_name_ref` = None and we are set to not to use the reference histograms"))
# In[11]:
# qa_file_new = ROOT.TFile.Open(qa_file_name_new);
# assert qa_file_new.IsOpen()
# qa_file_new.ls()
display(Markdown(f"Openning QA file at `{qa_file_name_new}`"))
ROOT.gInterpreter.ProcessLine(f"TFile *qa_file_new = new TFile(\"{qa_file_name_new}\");")
ROOT.gInterpreter.ProcessLine(f"const char * qa_file_name_new = \"{qa_file_name_new}\";")
if qa_file_name_ref is not None:
# qa_file_ref = ROOT.TFile.Open(qa_file_name_ref);
# assert qa_file_ref.IsOpen()
display(Markdown(f"Openning QA reference file at `{qa_file_name_ref}`"))
ROOT.gInterpreter.ProcessLine(f"TFile *qa_file_ref = new TFile(\"{qa_file_name_ref}\");")
ROOT.gInterpreter.ProcessLine(f"const char * qa_file_name_ref = \"{qa_file_name_ref}\";")
else:
ROOT.gInterpreter.ProcessLine(f"TFile *qa_file_ref = nullptr;")
ROOT.gInterpreter.ProcessLine(f"const char * qa_file_name_ref = nullptr;")
# In[12]:
get_ipython().run_cell_magic('cpp', '', '\nif (qa_file_new == nullptr) \n{\n cout <<"Error, can not open QA root file"<ls();\n\n//TFile *qa_file_ref = NULL;\n//if (qa_file_name_ref)\n//{\n// qa_file_ref = new TFile(qa_file_name_ref);\n// \n// if (qa_file_ref == nullptr) \n// {\n// cout <<"Error, can not open QA root file"<( qa_file_new->GetObjectChecked( Form( "%s%s_%i", prefix.Data(), tag.Data(), layer ), "TH1" ) );\n hnew->Scale( 1./hnew->GetEntries() );\n hnew->SetMinimum(0);\n\n // reference\n auto href = qa_file_ref ? static_cast( qa_file_ref->GetObjectChecked( Form( "%s%s_%i", prefix.Data(), tag.Data(), layer ), "TH1" ) ) : nullptr;\n if( href )\n {\n href->Scale( 1./href->GetEntries() );\n href->SetMinimum(0);\n }\n\n // draw\n cv->cd( ilayer+1 );\n DrawReference(hnew, href);\n\n auto line = VerticalLine( gPad, 0 );\n line->Draw();\n }\n\n return cv;\n\n}\n')
# # Azimuthal arc-length, $r \Delta\phi$, residuals, errors and pulls
# ## Residuals
# In[15]:
get_ipython().run_cell_magic('cpp', '', '\nDraw( qa_file_new, qa_file_ref, hist_name_prefix, "drphi" )-> Draw();\n')
# ## Errors
# In[16]:
get_ipython().run_cell_magic('cpp', '', '\nDraw( qa_file_new, qa_file_ref, hist_name_prefix, "rphi_error" )-> Draw();\n')
# ## Pulls
# In[17]:
get_ipython().run_cell_magic('cpp', '', '\nDraw( qa_file_new, qa_file_ref, hist_name_prefix, "phi_pulls" )-> Draw();\n')
# In[ ]:
# # $z$ residuals, errors and pulls
# ## Residuals
# In[18]:
get_ipython().run_cell_magic('cpp', '', '\nDraw( qa_file_new, qa_file_ref, hist_name_prefix, "dz" )-> Draw();\n')
# ## Errors
# In[19]:
get_ipython().run_cell_magic('cpp', '', '\nDraw( qa_file_new, qa_file_ref, hist_name_prefix, "z_error" )-> Draw();\n')
# ## Pulls
# In[20]:
get_ipython().run_cell_magic('cpp', '', '\nDraw( qa_file_new, qa_file_ref, hist_name_prefix, "z_pulls" )-> Draw();\n')
# # Cluster sizes
# ## Cluster size
# In[21]:
get_ipython().run_cell_magic('cpp', '', '\nDraw( qa_file_new, qa_file_ref, hist_name_prefix, "clus_size" )-> Draw();\n')
# ## Cluster azimuthal size
# In[22]:
get_ipython().run_cell_magic('cpp', '', '\nDraw( qa_file_new, qa_file_ref, hist_name_prefix, "clus_size_phi" )-> Draw();\n')
# ## Cluster $z$-size
# In[23]:
get_ipython().run_cell_magic('cpp', '', '\nDraw( qa_file_new, qa_file_ref, hist_name_prefix, "clus_size_z" )-> Draw();\n')
# In[ ]:
# # Summary statistics
# In[24]:
get_ipython().run_cell_magic('cpp', '', '\nKSTestSummary::getInstance()->make_summary_txt("QA-Intt.txt");\n')
# In[25]:
get_ipython().run_cell_magic('cpp', '', '\nKSTestSummary::getInstance()->make_summary_TCanvas() -> Draw();\n')