#!/usr/bin/env python
# coding: utf-8
# #
Pandas Datetime [25 exercises with solution]
# In[2]:
# Importing necessary libraries:
import numpy as np
import pandas as pd
# In[3]:
# Knowing about the csv file:
ufo_data = pd.read_csv('ufo.csv', index_col = 'Date_time', parse_dates = True)
ufo_data.head()
# 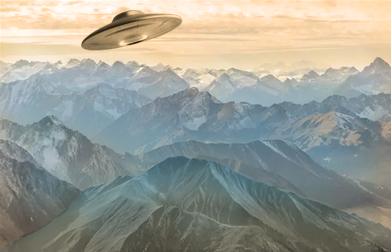
# 
# ## 25 Exercises Solutions
# ### 1. Write a Pandas program to create the todays date.
# In[4]:
from datetime import date
now = pd.to_datetime(date.today()) # '2019-11-02 00:00:00'
date = str(now).split()[0] # After split: ['2019-11-02', '00:00:00']
print('Today\'s Date->', date)
# ### 2. Write a Pandas program to calculate all the sighting days of the unidentified flying object (ufo) from current date.
# In[5]:
date_diff = (now - ufo_data.index).days # difference of corresponding time in days
result = date_diff.to_frame(index = False, name='Diff in Days')
print(result)
# ### 3. Write a Pandas program to get the current date, oldest date and number of days between Current date and oldest date of Ufo dataset.
# In[ ]:
# ### 4. Write a Pandas program to get all the sighting days of the unidentified flying object (ufo) which are less than or equal to 40 years (365*40 days).
# In[ ]:
# ### 5. Write a Pandas program to get all the sighting days of the unidentified flying object (ufo) between 1950-10-10 and 1960-10-10.
# In[ ]:
# ### 6. Write a Pandas program to get all the sighting years of the unidentified flying object (ufo) and create the year as column.
# In[ ]:
# ### 7. Write a Pandas program to create a plot to present the number of unidentified flying object (UFO) reports per year.
# In[ ]:
# ### 8. Write a Pandas program to extract year, month, day, hour, minute, second and weekday from unidentified flying object (UFO) reporting date.
# In[ ]:
# ### 9. Write a Pandas program to convert given datetime to timestamp.
# In[ ]:
# ### 10. Write a Pandas program to count year-country wise frequency of reporting dates of unidentified flying object(UFO).
# In[ ]:
# ### 11. Write a Pandas program to extract unique reporting dates of unidentified flying object (UFO).
# In[ ]:
# ### 12. Write a Pandas program to get the difference (in days) between documented date and reporting date of unidentified flying object (UFO).
# In[ ]:
# ### 13. Write a Pandas program to add 100 days with reporting date of unidentified flying object (UFO).
# In[ ]:
# ### 14. Write a Pandas program to generate sequences of fixed-frequency dates and time spans.
# In[ ]:
# ### 15. Write a Pandas program to create a conversion between strings and datetime.
# In[ ]:
# ### 16. Write a Pandas program to manipulate and convert date times with timezone information.
# In[ ]:
# ### 17. Write a Pandas program to get the average mean of the UFO (unidentified flying object) sighting was reported.
# In[ ]:
# ### 18. Write a Pandas program to create a graphical analysis of UFO (unidentified flying object) Sightings year.
# In[ ]:
# ### 19. Write a Pandas program to check the empty values of UFO (unidentified flying object) Dataframe.
# In[ ]:
# ### 20. Write a Pandas program to create a plot of distribution of UFO (unidentified flying object) observation time.
# In[ ]:
# ### 21. Write a Pandas program to create a graphical analysis of UFO (unidentified flying object) sighted by month.
# In[ ]:
# ### 22. Write a Pandas program to create a comparison of the top 10 years in which the UFO was sighted vs the hours of the day.
# In[ ]:
# ### 23. Write a Pandas program to create a comparison of the top 10 years in which the UFO was sighted vs each Month.
# In[ ]:
# ### 24. Write a Pandas program to create a heatmap (rectangular data as a color-encoded matrix) for comparison of the top 10 years in which the UFO was sighted vs each Month.
# In[ ]:
# ### 25. Write a Pandas program to create a Timewheel of Hour Vs Year comparison of the top 10 years in which the UFO was sighted.
# In[ ]:
# Data Sources: ufo_sighting_data.csv - [80,332 records]
# Exercises data sources: ufo.csv - [347 random records from ufo_sighting_data.csv]
#
# Note there are no missing data in the columns (ufo.csv).
#
# Source:
# - https://github.com/planetsig/ufo-reports
# - https://www.kaggle.com/camnugent/ufo-sightings-around-the-world
# #### Reference: [https://www.w3resource.com/python-exercises/pandas/datetime/index.php](https://www.w3resource.com/python-exercises/pandas/datetime/index.php)